Tapfiliate Stripe Checkout JS integration with Wix Corvid
UPDATE 06 April 2021 : We are quite deceived by Tapfiliate because it does not support sending payouts from Tapfiliate. We do not see the interest of paying $70/mo to do it manually every month. We decided to implement our own solution.
Tapfiliate does not work out of the box with stripe checkout (client side only) and wix.
Keep in mind that this is a little bit advanced so you will need to be familiar with Wix, Javascript (node) and Stripe API.
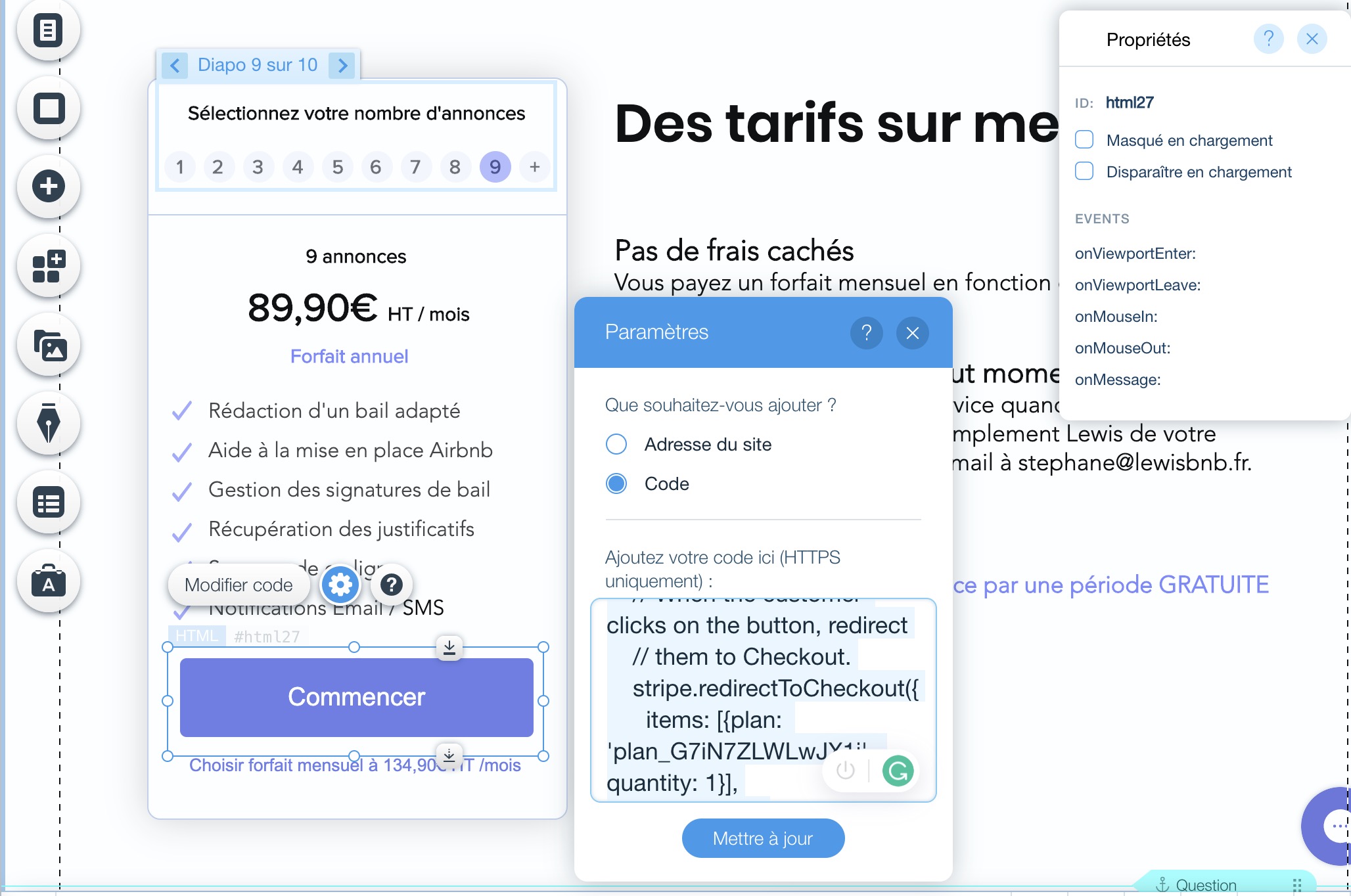
My use case is classic SaaS business model : customers have a 15 days trial then pay monthly or annually.
The officials integrations does not work for me :
- https://tapfiliate.com/docs/integrations/wix/
=> I am not using wix store payments - https://tapfiliate.com/docs/integrations/stripe/
=> I don't have access to customer_id from client side stripe checkout
Main problems I faced :
- I don't have access to stripe
customer_id
without running server-side code tap
have to run within the page and not js page code or html element because of cookies scope
Here I will explain how I successfully setup Tapfiliate with Stripe all within Wix corvid without any external dependencies :
- Configure Stripe Checkout button to return a
session_id
- Enable Wix Corvid (developer mode)
- Fetch
customer_id
from Stripe API using wix back-end code - Redirect to same page with
?customer_id=cus_xxxxx
- Use that URL parameter to
tap('trial', cus_xxxxxx)
Configure Stripe Checkout to return session_id
Unfortunately Stripe does not return a customer_id
on successful payment or even trial.
First thing to do is to create/update your checkout js success_url
to return a session_id
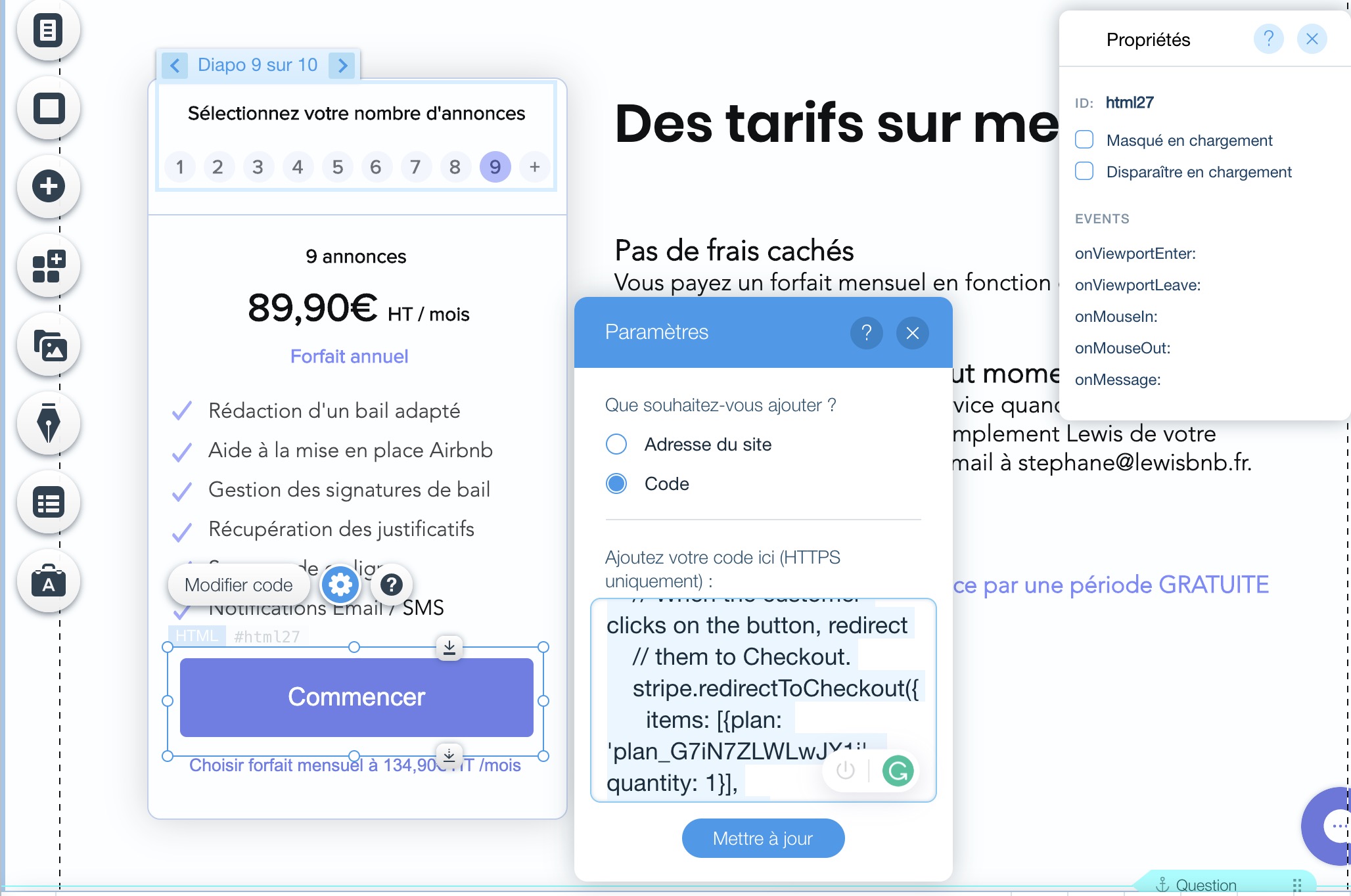
HTML element
on a wix page<!-- Load Stripe.js on your website. -->
<script src="https://js.stripe.com/v3"></script>
<!-- Create a button that your customers click to complete their purchase. Customize the styling to suit your branding. -->
<button
style="background-color:#6772E5;color:#FFF;padding:19px 82px;border:0px solid rgb(127, 0, 0);border-radius:5px;border-color:#6772E5;font-size:1.2em;cursor:pointer"
onmouseover="this.style.backgroundColor='#0a0a29';this.style.borderColor='#0a0a29;'"
onmouseout="this.style.backgroundColor='#6772E5';this.style.borderColor='#6772E5;';return true;"
id="checkout-button-plan_G7iN7ZLWLwJX1i"
role="link"
>
Commencer
</button>
<div id="error-message"></div>
<script>
(function() {
var stripe = Stripe('pk_live_my-key');
var checkoutButton = document.getElementById('checkout-button-plan_id');
checkoutButton.addEventListener('click', function () {
// When the customer clicks on the button, redirect
// them to Checkout.
stripe.redirectToCheckout({
items: [{plan: 'my-plan-id', quantity: 1}],
locale: "fr",
// Do not rely on the redirect to the successUrl for fulfilling
// purchases, customers may not always reach the success_url after
// a successful payment.
// Instead use one of the strategies described in
// https://stripe.com/docs/payments/checkout/fulfillment
successUrl: 'https://www.lewisbnb.fr/success?session_id={CHECKOUT_SESSION_ID}',
cancelUrl: 'https://www.lewisbnb.fr/canceled',
})
.then(function (result) {
if (result.error) {
// If `redirectToCheckout` fails due to a browser or network
// error, display the localized error message to your customer.
var displayError = document.getElementById('error-message');
displayError.textContent = result.error.message;
}
});
});
})();
</script>
π Complete script
Now that Stripe returns a session_id you will be able to get a customer_id
with server-side code.
Enable Wix Corvid
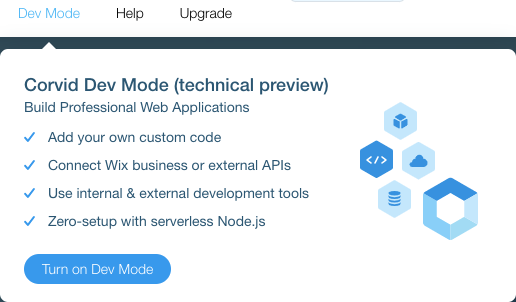
This mode is for developer but it is awesome, you can :
- run backend node js code like 3rd party API calls...
- run front end code like updating the code
Everything in ES6.
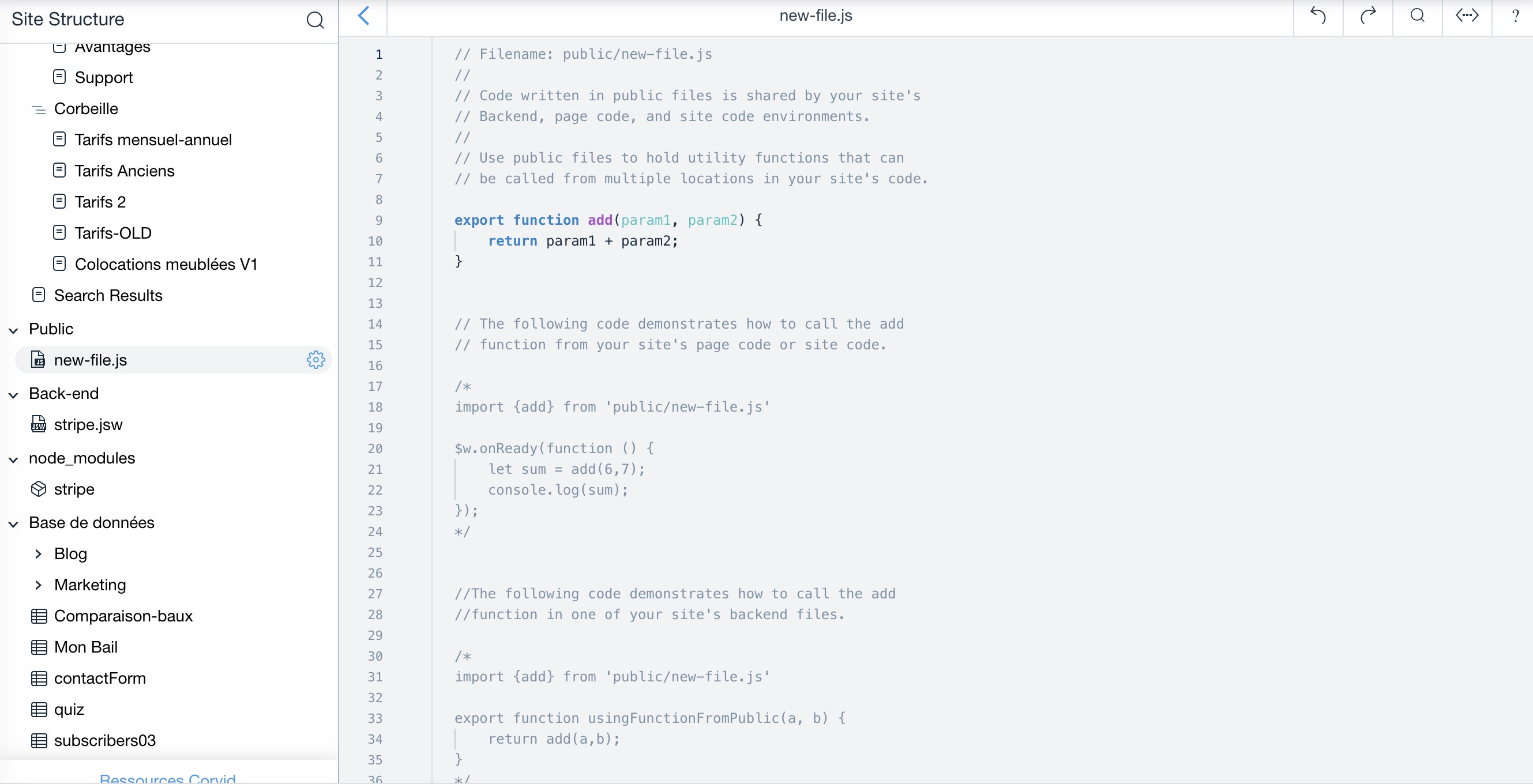
Setup a thank you page
We want to fetch our customer_id
by using the session_id
returned by stripe on successful payment.
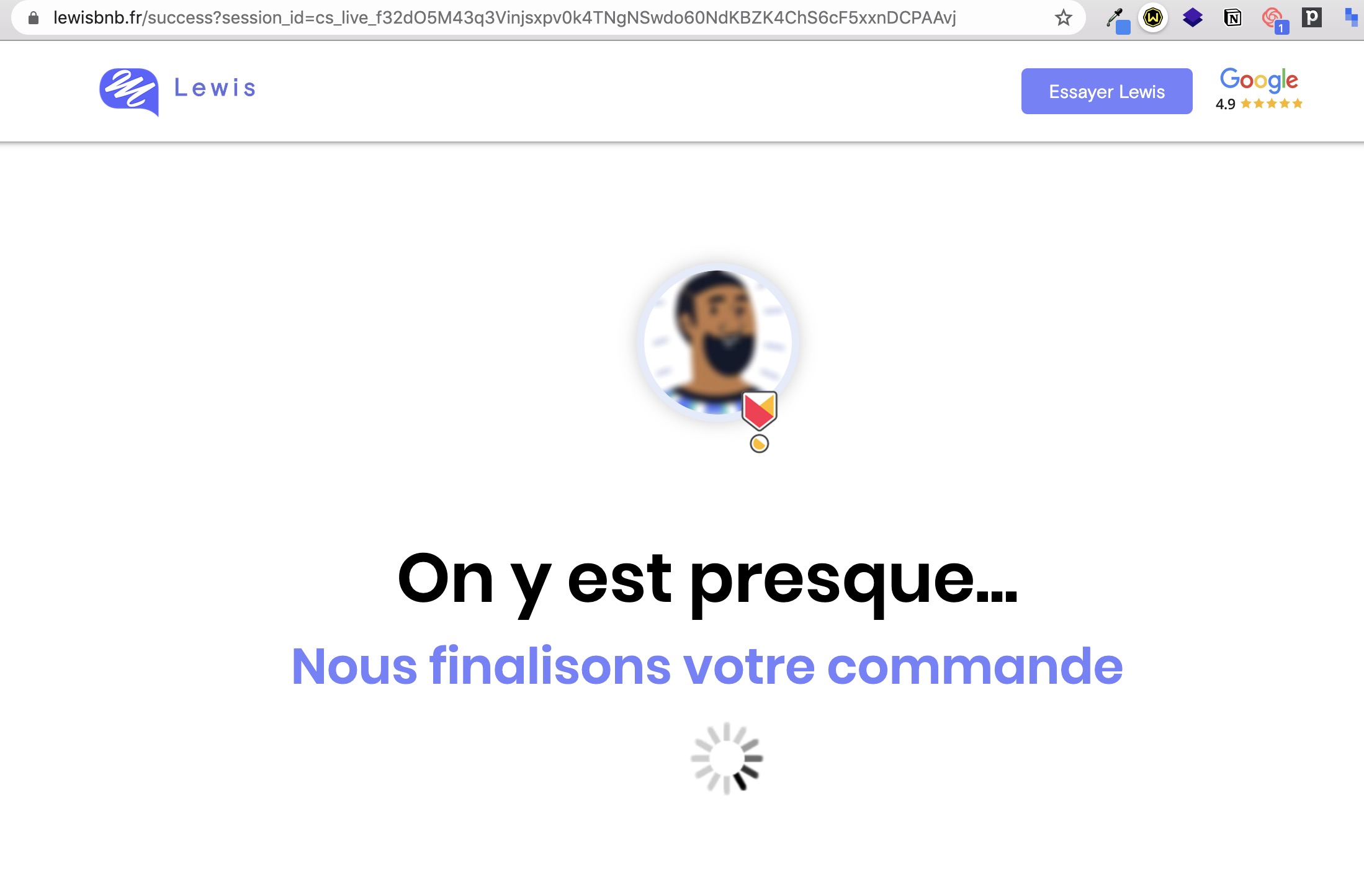
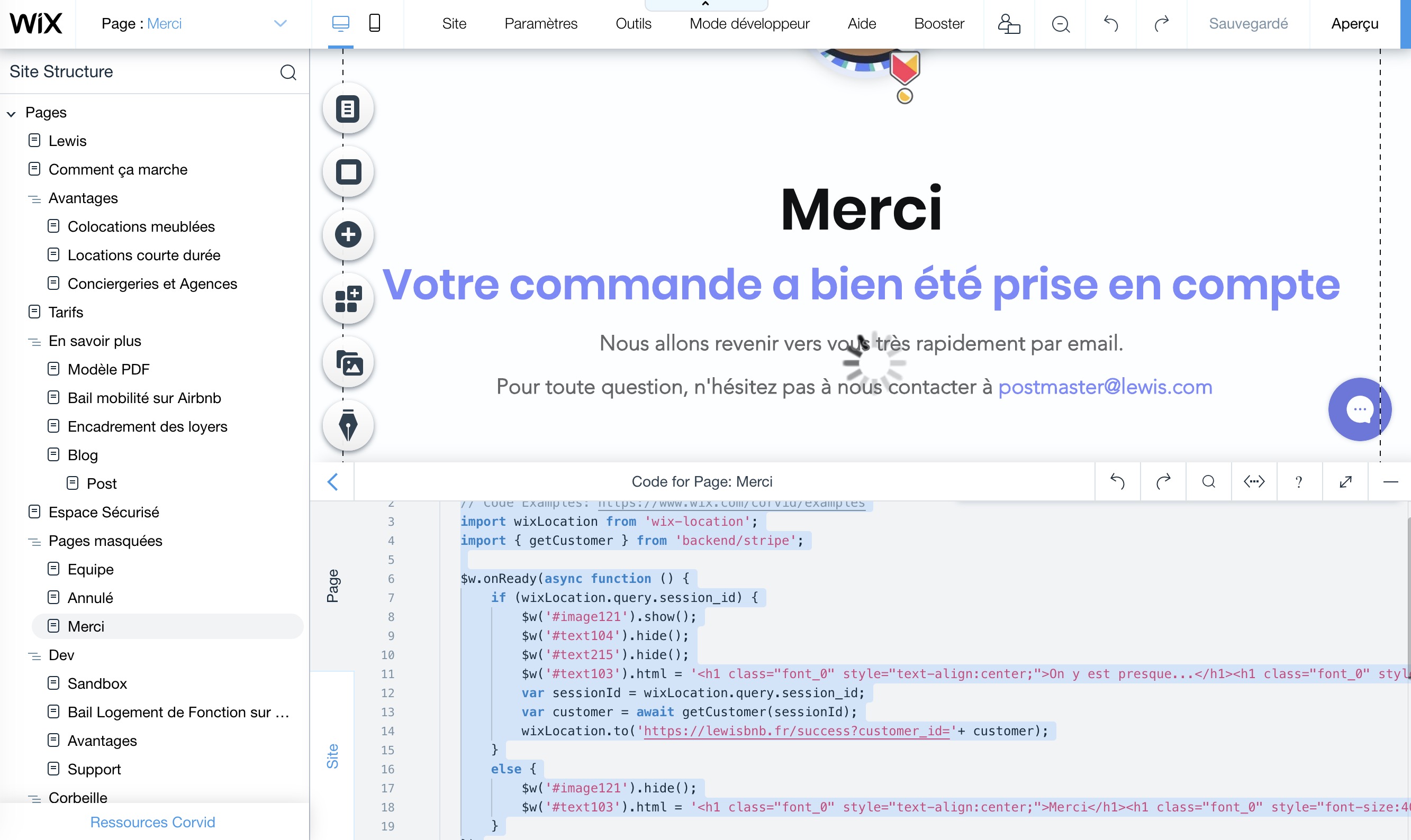
/success?customer_id=cus_xxx
Full code:
// API Reference: https://www.wix.com/corvid/reference
// Code Examples: https://www.wix.com/corvid/examples
import wixLocation from 'wix-location';
import { getCustomer } from 'backend/stripe';
$w.onReady(async function () {
if (wixLocation.query.session_id) {
$w('#image121').show();
$w('#text104').hide();
$w('#text215').hide();
$w('#text103').html = '<h1 class="font_0" style="text-align:center;">On y est presque...</h1><h1 class="font_0" style="font-size:40px; text-align:center;"><span style="font-size:40px;"><span style="color:#777DFC;">Nous finalisons votre commande</span></span></h1></div>';
var sessionId = wixLocation.query.session_id;
var customer = await getCustomer(sessionId);
wixLocation.to('https://lewisbnb.fr/success?customer_id='+ customer);
}
else {
$w('#image121').hide();
$w('#text103').html = '<h1 class="font_0" style="text-align:center;">Merci</h1><h1 class="font_0" style="font-size:40px; text-align:center;"><span style="font-size:40px;"><span style="color:#777DFC;">Votre commande a bien Γ©tΓ© prise en compte</span></span></h1></div>';
}
});
What we just did :
- Hide/show some waiting text / spinner
- fetch
session_id
that we send togetCustomer
(defined in the backend next step) - Redirect to
/success?customer_id=cus_xxx
Stripe API in Corvid Wix back-end
- Install the Stripe package in
node_modules
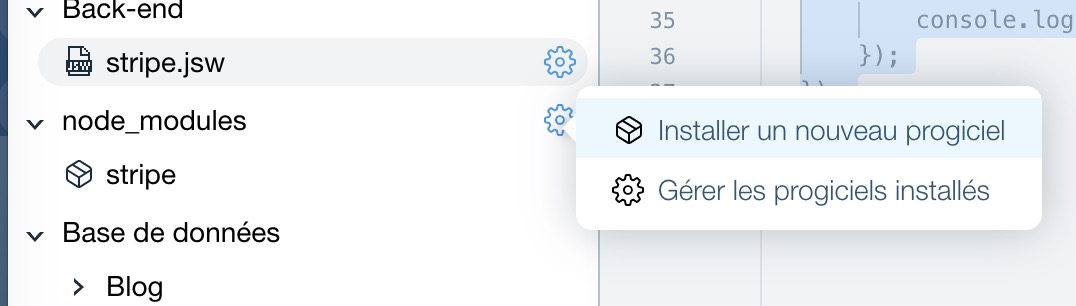
2. Add a stripe.jsw
file in Back-end and write the following code :
import Stripe from 'stripe';
// Filename: backend/stripe.jsw (web modules need to have a .jsw extension)
export async function getCustomer(sessionId) {
console.log('fetching customer....', sessionId);
try {
const stripe = new Stripe('rk_live_my-id');
const session = await stripe.checkout.sessions.retrieve(sessionId);
console.log("session-------", session);
return session.customer;
} catch(error) {
console.log(error);
return error;
}
}
Here we fetch the session using our Stripe API key. The API call is defined there :
Add Tapfiliate script in all the pages
Go to Parameters > Monitoring and Analytics
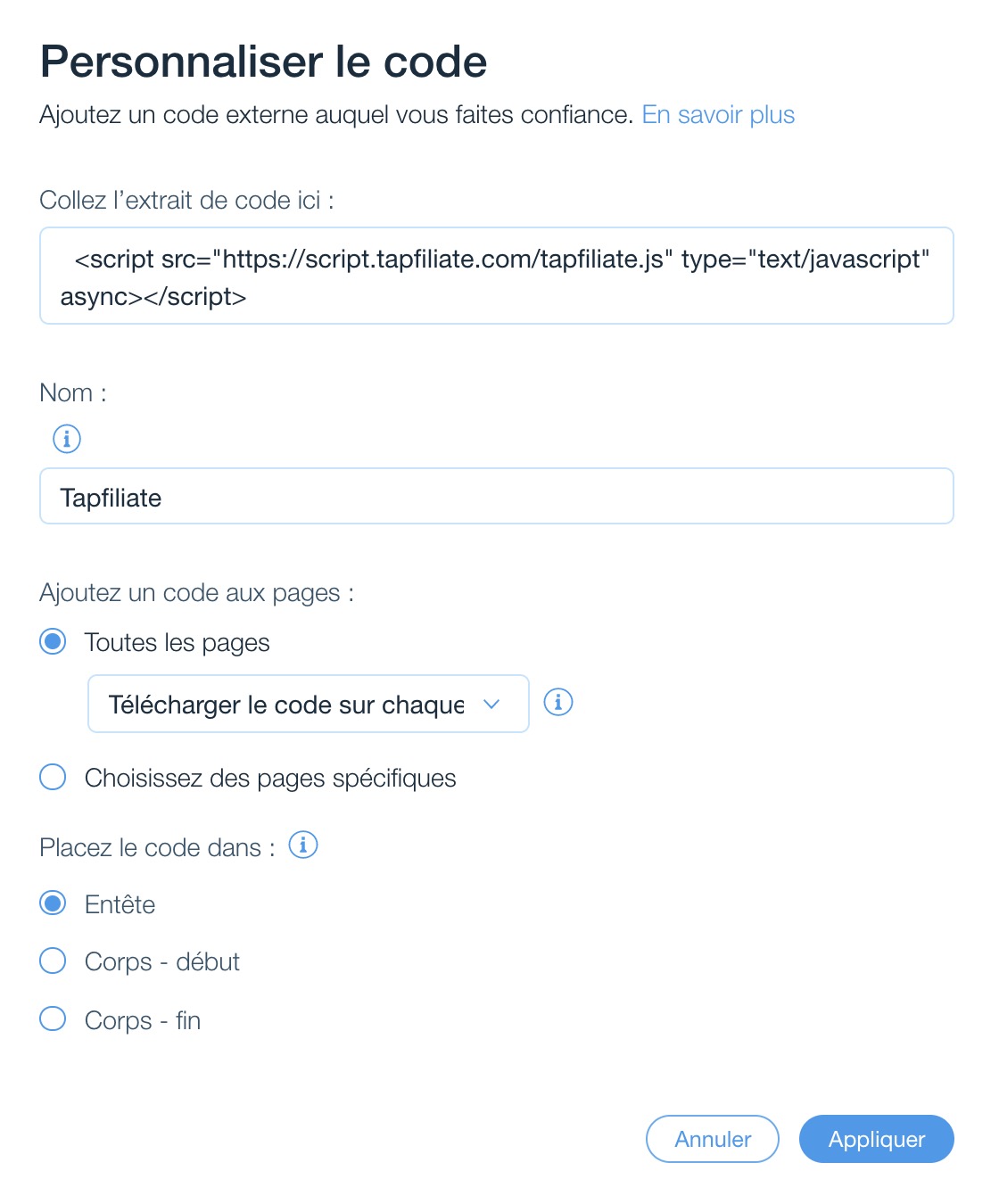
New Tools > Custom
<script src="https://script.tapfiliate.com/tapfiliate.js" type="text/javascript" async></script>
<script type="text/javascript">
function getQueryParams(){var r={},n=function(r){return decodeURIComponent(r.replace(/\+/g," "))},t=location.search.substring(1).split("&");for(var e in t){var o=t[e].toString().split("=");o.length>1&&(r[n(o[0])]=n(o[1]))}return r}
(function(t,a,p){t.TapfiliateObject=a;t[a]=t[a]||function(){
(t[a].q=t[a].q||[]).push(arguments)}})(window,'tap');
tap('create', 'my-tapfiliate-account-id', { integration: "stripe" });
tap('detect');
var params = getQueryParams();
console.log(params);
if (params.customer_id) {
tap('trial', params.customer_id);
}
</script>
What this does :
- detect if there are
ref=....
and set cookies - if there are any
customer_id
it will set astrial
on customer
Make sure this whole thing work you will need to create an affiliate and test everything
Conclusion
I would prefer to rely on webhooks rather than a thankyou
page for security reasons.
In my case any customers are trial first then they are converted on Stripe only when their trial expires so I believe this won't an issue. I hope.